Routing | Next.js Supabase SaaS Kit
Learn the basics of routing in the Next.js Supabase SaaS Boilerplate
Before getting started with building our Tasks Pages, let's take a look at the default page structure of the boilerplate:
├── app └── layout.tsx └── onboarding (site) └── faq └── pricing └── auth └── link └── password-reset └── sign-in └── sign-up └── invite └── [code] └── page.tsx └── dashboard └── [organization] └── page.tsx └── settings └── organization └── members └── page.tsx └── invite └── page.tsx └── profile └── index └── email └── password └── authentication └── subscription └── page.tsx
The routes are split in the following way:
- The website's pages are placed under
(site)
- The auth pages are placed under
auth
- The internal pages (behind auth) that are specific to organizations are placed under
dashboard/[organization]
- Some pages in the "middle" are placed outside
dashboard/[organization]
, such as the invites page and the onboarding flow.
Setting the application's Home Page
By default, the application's home page is /dashboard/[organization]
; every time the user logs in, they're redirected to the page src/app/dashboard/[organization]/page.tsx
.
The path dashboard
is also defined as appPrefix
in the configuration file src/configuration.tsx
: you can update this and rename the folder if you want to change the path.
To update the application's home page, you can update the configuration.paths.appHome
variable in src/configuration.tsx
.
Demo: Tasks Application
Our demo application will be a simple tasks management application. We will be able to create tasks, list them, mark them as completed, and delete them.
Here is a quick demo of what we will build:
Loading video...
Routing
Ok, so we want to add three pages to our application:
- Tasks List: A page to list all our tasks
- Task Detail: A page specific to the selected task
To create these two pages, we will create a folder named tasks
at src/app/dashboard/[organization]/tasks
.
In the folder src/app/dashboard/[organization]/tasks
we will create three Page Components
:
- The tasks list page at
tasks/page.tsx
- The task's own detail page at
tasks/[task]/page.tsx
.
├── app └── dashboard/[organization] └── tasks └── page.tsx └── [task] └── page.tsx
Adding Functionalities to your application
To add new functionalities to your application (in our case, tasks management), usually, you'd need the following things:
- Data Model: first, we want to define the data model of the feature you want to add
- Supabase Queries/Mutations: once we're happy with the data model, we can create our Supabase DB queries/mutations
- Build Feature components: then we can build the components of the feature (forms, lists, etc.)
- Add feature components into the pages: finally, we add the components to the pages
Adding page links to the Navigation Menu
Updating the Top header Navigation
To update the navigation menu, we need to update the NAVIGATION_CONFIG
object in src/navigation-config.tsx
. This object contains the links to the pages that are displayed in the top header menu.
- The
label
is the text that will be displayed in the menu - it's a translation key that you can find inlocales/[lang]/common.json
- The
path
is the path to the page
const NAVIGATION_CONFIG = (organization: string) => ({ items: [ { label: 'common:dashboardTabLabel', path: getPath(organization, ''), Icon: ({ className }: { className: string }) => { return <Squares2X2Icon className={className} />; }, end: true, }, { label: 'common:tasksTabLabel', path: getPath(organization, 'tasks'), Icon: ({ className }: { className: string }) => { return <RectangleStackIcon className={className} />; }, }, { label: 'common:settingsTabLabel', path: getPath(organization, 'settings'), Icon: ({ className }: { className: string }) => { return <Cog8ToothIcon className={className} />; }, }, ],});function getPath(organizationId: string, path: string) { const appPrefix = configuration.paths.appPrefix; return [appPrefix, organizationId, path].filter(Boolean).join('/');}
To add a new link to the header menu, follow the same convention:
- Add a new item to the
items
array - Add the
label
andpath
properties - Make sure the
label
is a translation key that you can find inlocales/[lang]/common.json
- Add the
Icon
property if you want to display an icon next to the label - Make sure the
path
is correct
The result will be similar to the images below:
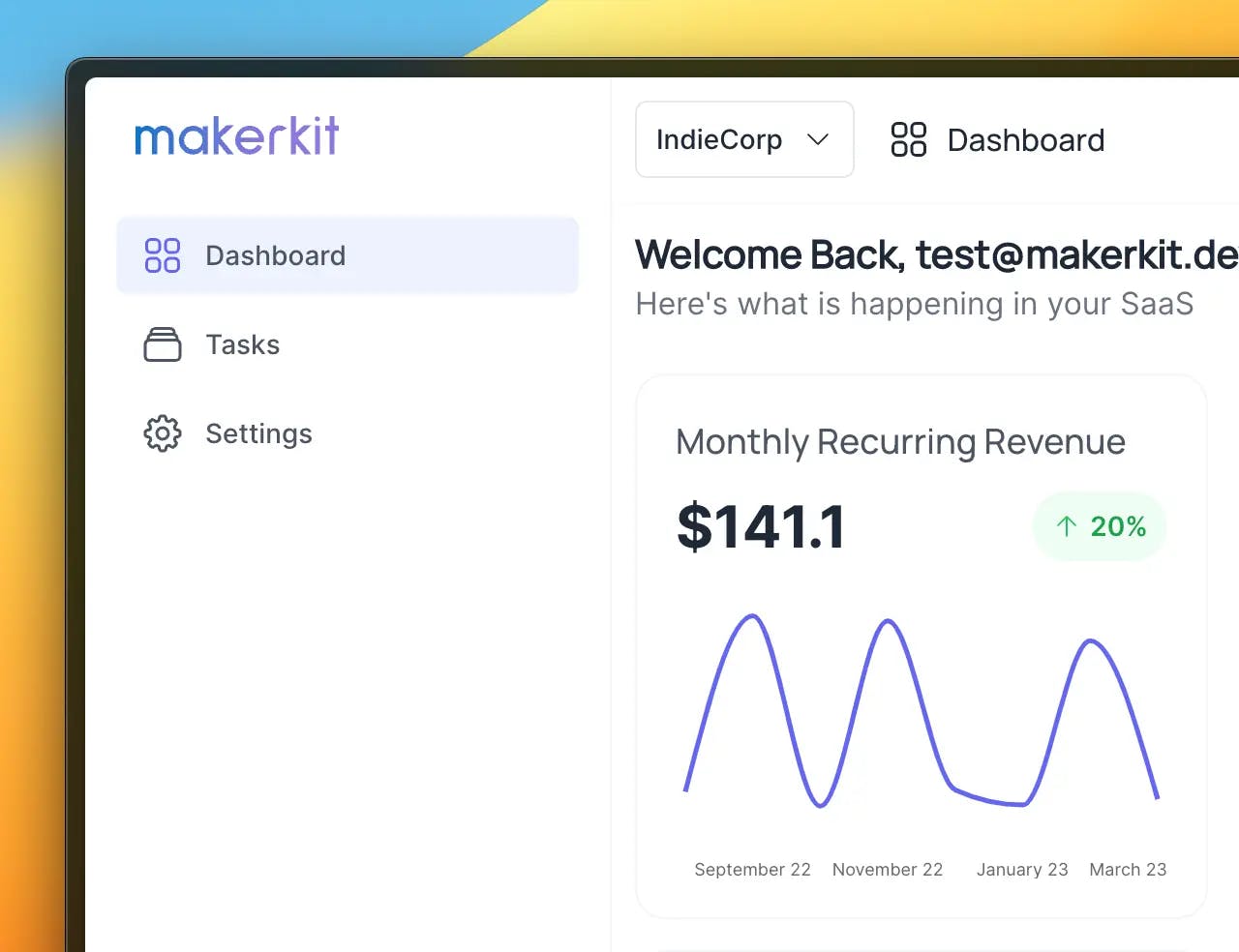
In the next sections we will go into more detail about how to use and configure Application Pages.