Next.js is one of the most popular JavaScript frameworks for building web applications. It has a lot of built-in functionality that allows developers to build full-featured applications with React.
Google Analytics is the world's most powerful web analytics platform, used by millions of websites and businesses to track user behavior and measure conversions. When combined, they provide you with a powerful combination for tracking data about your user base — letting you see what parts of your application users are using most often, which pages they're visiting, etc...
In this post, I will show you how easy it is to get NextJS and Google Analytics working together.
How to set up Next.js to use Google Analytics
The first step to integrating Google Analytics is setting up a Google Analytics account and creating a property. Create an account on the Google Analytics website, navigate to the Admin tab and click on "Tracking Info."
If you are creating a Firebase project and you decided to create a Google Analytics property as part of it, you will find the Analytics Tracking Code in your settings. This is also called "Measurement ID".
Adding Google Analytics with reactfire
If you are using the library reactfire
, an official library by Firebase that helps you work more easily with Google Analytics, the process is straightforward.
The only thing we need to do, if we're using SSR, is to call the component conditionally and only instantiate it on the client side. In fact, this component does not work on the server, and it doesn't make much sense anyway.
import { getAnalytics } from 'firebase/analytics';import { AnalyticsProvider } from 'reactfire';import { isBrowser } from '~/core/generic';const FirebaseAnalyticsProvider: React.FCC = ({ children }) => { const sdk = isBrowser() ? getAnalytics() : undefined; if (!sdk) { return <>{children}</>; } return <AnalyticsProvider sdk={sdk}>{children}</AnalyticsProvider>;};export default FirebaseAnalyticsProvider;
Have you noticed we haven't defined the tracking code anywhere? This is because the SDK will automatically detect it from the main application's configuration that we pass to FirebaseAppProvider
to create the Firebase SDK on page.
Below in the tree (important, because we need access to the SDK providers), we will use another component to initialize the tracking:
function AnalyticsTrackingEventsProvider({ children,}: React.PropsWithChildren) { useAnalyticsTracking(); return <>{children}</>;}
What does useAnalyticsTracking
do? In this hook, we add all the initial hooks to start tracking at page load, such as the ID of the user, the screen views and so on.
function useAnalyticsTracking() { useTrackSignedInUser(); useTrackScreenViews();}
Tracking signed-in users with Google Analytics
One of the most important parts to segmentation is knowing if the user is signed-in or not. To do this, we have to check if the current signed in users is identified by the Firebase SDK. If so, we track it with Google Analytics, that will then be able to create segments based on this factor.
Retrieving the User ID
Depending on the type of authentication you use, the below may look very different. If you have an application bootstrapped with Makerkit, it will be fairly simple:
function useCurrentUserId() { const user = useUserSession(); return user?.auth?.uid;}
Setting the user ID
Now, we can call the setUserId
function imported from firebase/analytics
:
import { getAnalytics, setUserId } from 'firebase/analytics';export function useTrackSignedInUser() { const userId = useCurrentUserId(); useEffect(() => { if (!isBrowser()) { return; } const analytics = getAnalytics(); if (userId) { setUserId(analytics, userId); } }, [userId]);}
Because the function getAnalytics
will crash your application on the server, we make sure to only call it on the client side.
Tracking page views
To track page views, we need to use the Next.js router and listen to views changes so we can intercept client-side navigations.
Additionally, we use logEvent
, a function from firebase/analytics
that allows us to send events to Google Analytics (as if we were directly using the dataLayer
).
import { useCallback, useEffect } from 'react';import { getAnalytics, logEvent } from 'firebase/analytics';import Router from 'next/router';function useTrackScreenViews() { const onRouteChangeComplete = useCallback(() => { if (!isBrowser()) { return; } const analytics = getAnalytics(); const title = document.title; logEvent(analytics, 'screen_view', { firebase_screen: title, firebase_screen_class: title, }); }, []); useEffect(() => { Router.events.on('routeChangeComplete', onRouteChangeComplete); return () => { Router.events.off('routeChangeComplete', onRouteChangeComplete); }; }, []);}
You can use logEvent
for sending both predefined and custom events.
Separating Website users from Application users
One of the challenges of hosting the application and the marketing website within the same domain and application is the fact that analytics will aggregate both visitors and users. To make this more manageable, we can create segments within Google Analytics, helping us understand the data for different types of users.
From your Google Analytics account, visit "Configure"->"Audiences". From here, we can create custom audiences to use as segments. In fact, we can restrict or aggregate our reports based on the selected audience.
From here, we can create two audiences:
- Visitors, ie. users who are browsing without being signed in
- Users, ie. users who have been identified and are signed-in
We can create a new audience, and select as a condition that the users are signed in, like below:
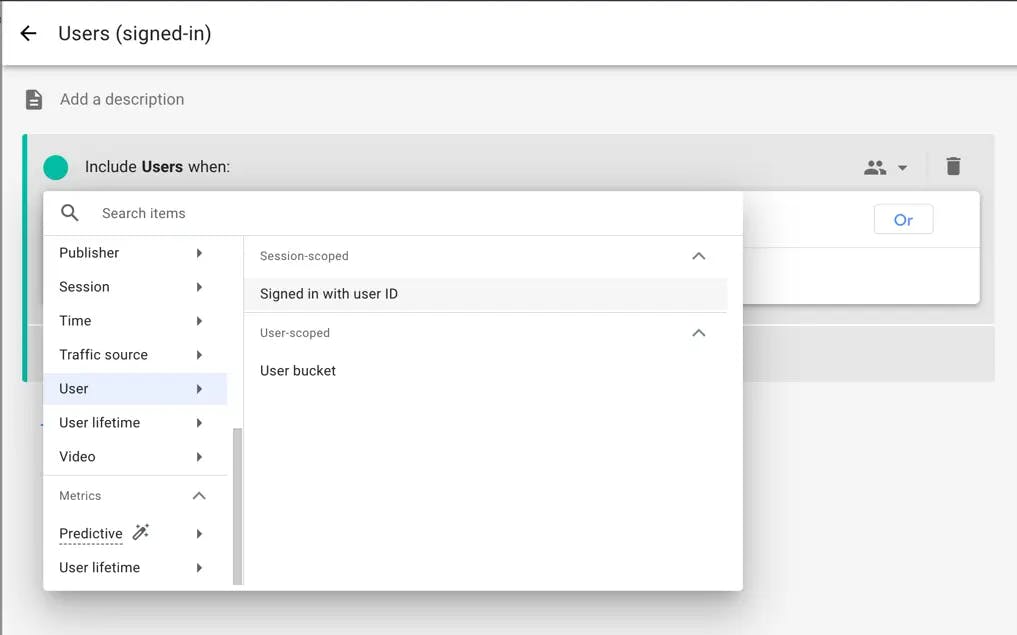
To create the "Visitors" audience, we simply need to negate the condition above using the logic builder.
Conclusion
Integrating Google Analytics with NextJS is a great way to get more insight into your website's analytics, such as how your users use the application, which types of users, and how they reach your website.
We hope this guide has helped you get started with Google Analytics and NextJS!