Firebase is a cloud backend platform backed by Google Cloud. Because it's a cloud environment, it wasn't possible to run it locally for a long time, which was rather complex and inconvenient during the development phase.
In addition, it required an Internet connection, and it was using the production services (even if it was a staging project) for just about anything, eating into the free quota available.
In 2021, Firebase finally finalized the roll-out of the Firebase Suite emulated locally. Installed as an NPM package, the firebase-tools
library allows us to spin up the emulator for each part of the Firebase Stack:
- Authentication
- Firestore
- RealTime DB
- Hosting
- Cloud Functions
- Cloud Storage
- Cloud PubSub
Furthermore, the Firebase Emulators come with a UI console similar to the actual Firebase Console, although much more limited.
This blog post explains how to set up the Firebase Emulators locally and integrate them into a Next.js application using reactfire
, the official Firebase package for React.
If you haven't created a Next.js application already, please run the following command:
npx create-next-app@latest --ts# oryarn create next-app --ts
Installing the Firebase Tools package
First, we need to install the firebase-tools
package for NodeJS. It's the Firebase CLI, which we can use for various tasks:
- switching projects
- install and run the emulators
- deploy the project
To install it in your existing Next.js project, please run the following command in your terminal, which installs the package globally:
npm i firebase-tools -g
In addition, please install the following NPM dependencies, handy to work with React and Firebase:
npm i -d reactfire firebase
Installing the Firebase Emulators
If you haven't created a Firebase project yet, please run the following command to initialize it:
firebase init
The command above should return the following output:
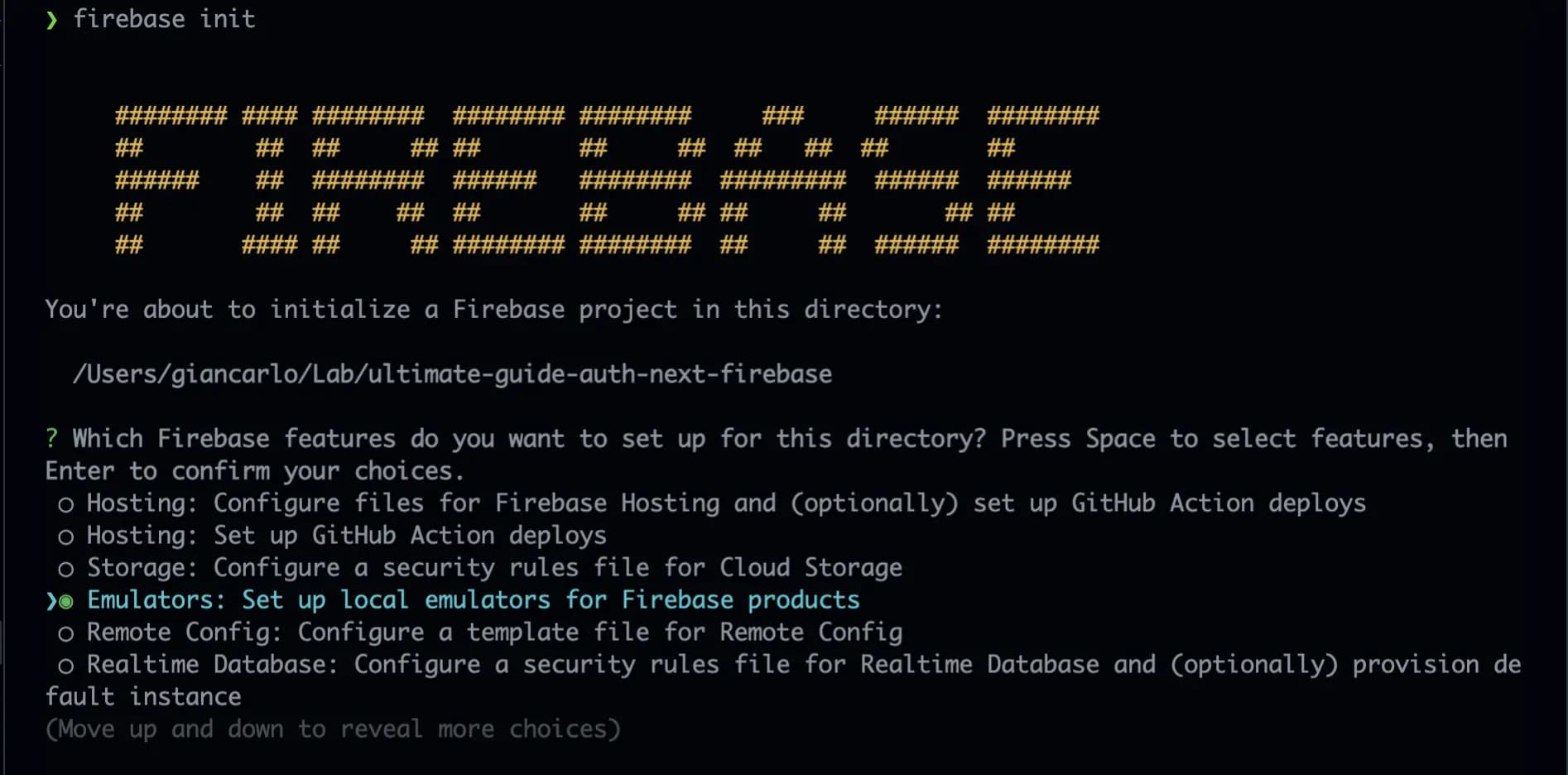
We recommend choosing the following options:
- Emulators
- Firestore
- Storage
Afterward, you can choose to create a new project, choose an existing one, or neither of them:
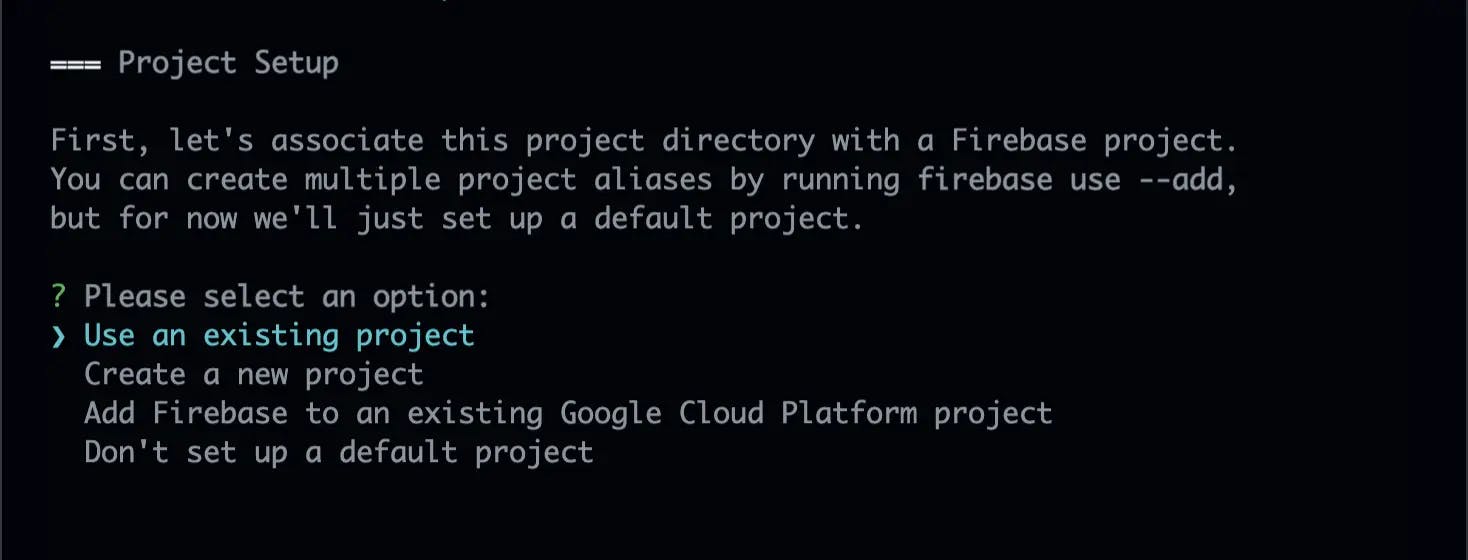
You are not required to create a project unless you use the Storage emulators.
The CLI should output the emulators you want to install: if you're unsure which ones you want, select them all except for Realtime DB and Cloud Functions. As you're using NextJS, we assume you want to use Vercel's API functions:
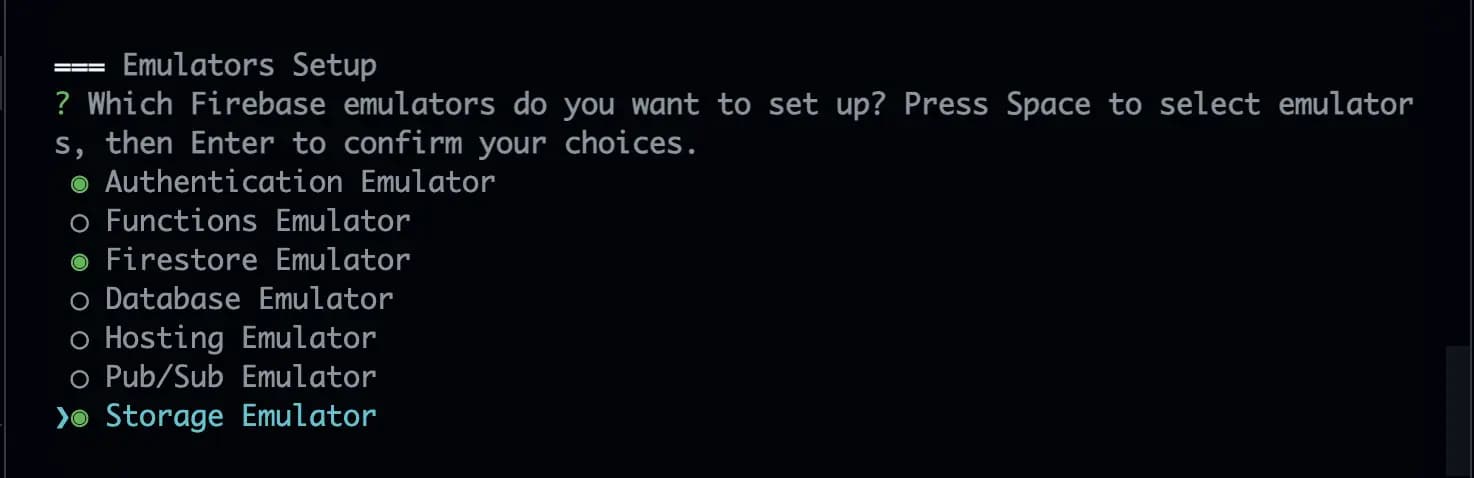
Finally, choose the ports assigned to each emulator and download the emulators. If you choose a port different from the default one, remember to update the Next.js environment variable we define in the next section.
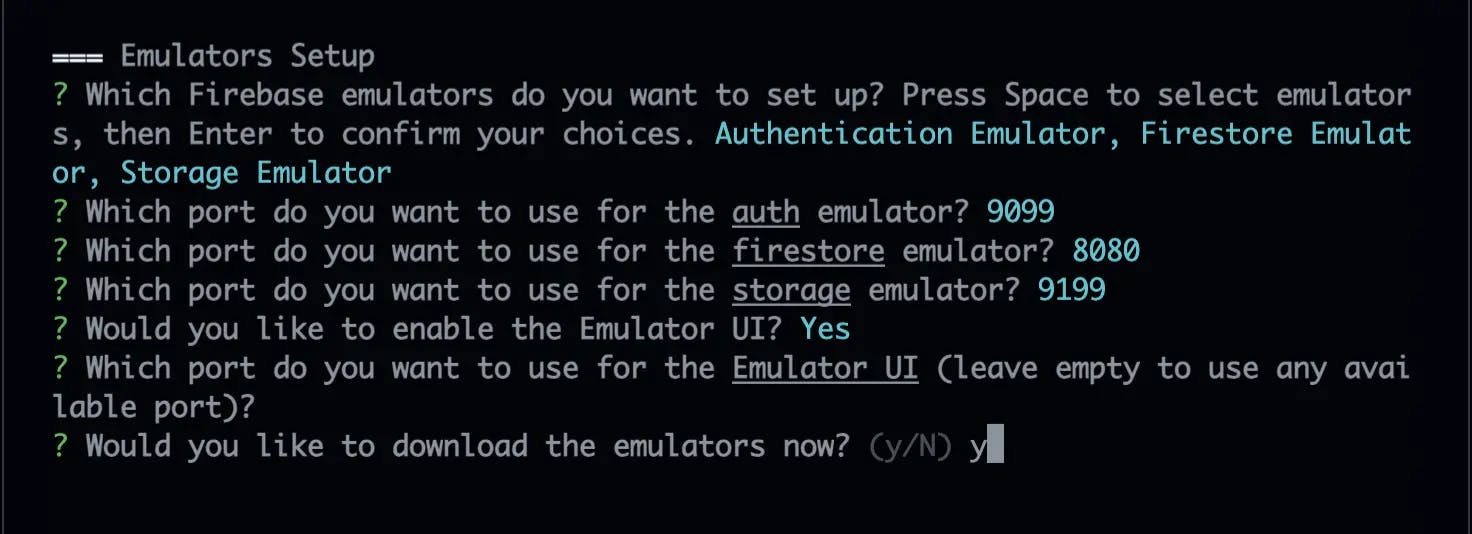
In case you have not yet created a Firebase project
If you have opted to start without creating a Firebase project, make sure to add a default project to your .firebaserc
file, even if it is non-existent yet.
Afterward, open .firebaserc
and paste the following content:
{ "projects": { "default": "ultimate-guide-auth-firebase-next" }}
NB: make sure to update the default project when you create one.
After the Installation Completes
After this command completes, your project is going to have the following files generated for you:
- .firebaserc- firebase.json- firestore.rules- firestore.indexes.json
NB: if you are running the Storage Emulators, Firebase does require you to select a project.
Adding the Emulators to the Next.js App
We can now configure our Next.js application to use the Emulators instead of the production Firebase services as we have the emulators installed.
We add the emulators' host and ports to the Next.js environment file. Do not use a production .env
file, but ideally use the local one (for example, .env
or .env.local
):
NEXT_PUBLIC_FIREBASE_EMULATOR_HOST=localhostNEXT_PUBLIC_EMULATOR=trueNEXT_PUBLIC_FIRESTORE_EMULATOR_PORT=8080NEXT_PUBLIC_FIREBASE_AUTH_EMULATOR_PORT=9099NEXT_PUBLIC_FIREBASE_STORAGE_EMULATOR_PORT=9199
Because we prefixed the environment variables with NEXT_PUBLIC_
, Webpack compiles and adds these to the final client-side bundle.
Make sure you choose the exact emulator ports we defined when installing the emulators. If you have not changed the default ports, you're all set!
Running the Emulators
To run the Firebase Emulators, we suggest creating a new NPM script that runs the following command:
{ "scripts": { "firebase:emulators:start": "firebase emulators:start" }}
Assuming everything went well, you should see the output below:
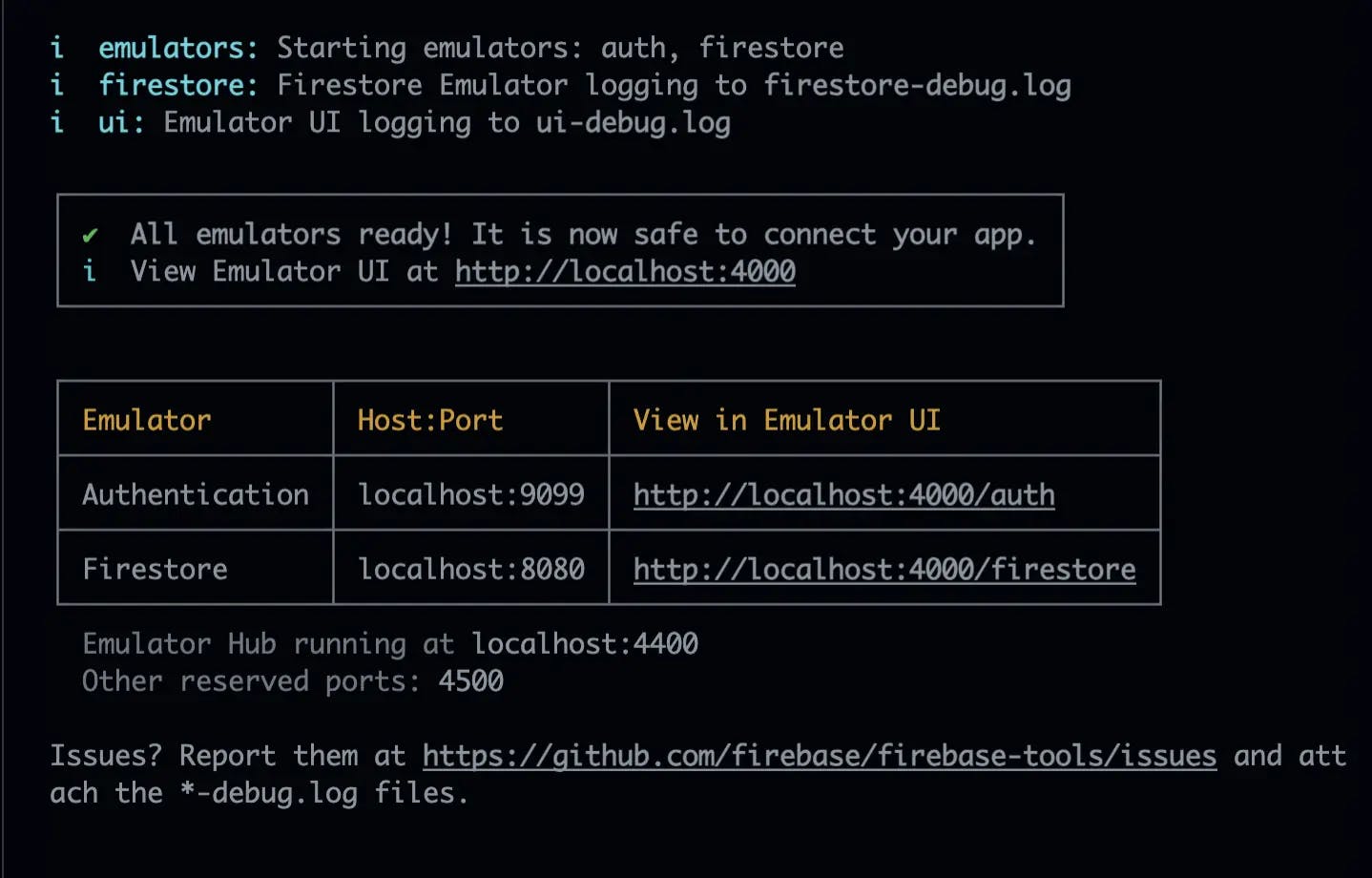
Yay! You did it!
Importing an Emulator Snapshot
To import a snapshot of the state, we need to add the parameter import
:
firebase emulators:start --import ./firebase-seed
You can change the directory path to something else if you want to.
Exporting the current Emulator snapshot
To export the current emulator's snapshot, we can add and run the following command:
{ "scripts": { "firebase:emulators:export": "firebase emulators:export ./firebase-seed" }}
Adding the Auth Emulator
To add the auth
emulator, we can import the function connectAuthEmulator
from the package firebase/auth
.
Let's create a component that connects to the Auth emulators and makes the SDK available to all its children:
import React, { useEffect } from 'react';import { AuthProvider, useAuth, useFirebaseApp } from 'reactfire';import { initializeAuth, indexedDBLocalPersistence, connectAuthEmulator, inMemoryPersistence,} from 'firebase/auth';function getAuthEmulatorHost() { // we can access these variables // because they are prefixed with "NEXT_PUBLIC_" const host = process.env.NEXT_PUBLIC_FIREBASE_EMULATOR_HOST; const port = process.env.NEXT_PUBLIC_FIREBASE_AUTH_EMULATOR_PORT; return ['http://', host, ':', port].join('');}function isBrowser() { return typeof window !== 'undefined';}export default function FirebaseAuthProvider({ children,}: React.PropsWithChildren) { const app = useFirebaseApp(); // make sure we're not using IndexedDB when SSR // as it is only supported on browser environments const persistence = isBrowser() ? indexedDBLocalPersistence : inMemoryPersistence; const sdk = initializeAuth(app, { persistence }); const shouldConnectEmulator = process.env.NEXT_PUBLIC_EMULATOR ==='true'; if (shouldConnectEmulator) { const host = getAuthEmulatorHost(); connectAuthEmulator(sdk, host); } return ( <> {children} </> );}
Whenever we want to use the Firebase Auth SDK within our application, we will need to wrap the page with the component defined above:
const PageWithAuth = () => { return ( <FirebaseAuthProvider> { // your page goes here } </FirebaseAuthProvider> );};export default PageWithAuth;
Are you looking for an example containing the code above? We got you sorted. The full source code is available on Github: it's an open-source codebase with a fully functioning Authentication system with Firebase and Next.js. Feel free to clone, extend and share it as much as you wish!
Check out our Ultimate Authentication Guide with Next.js and Firebase that explains in detail how we built the example above.